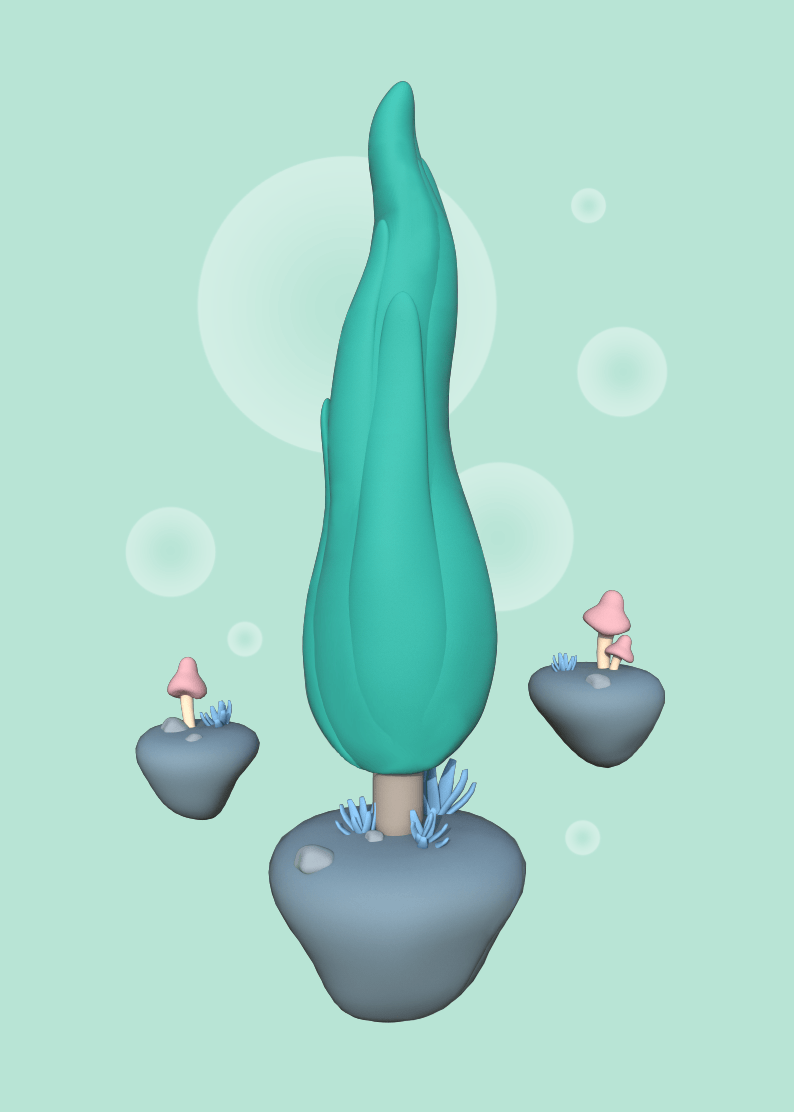
Cypress tests organizing (or any e2e/ui tests)
Cypress tests folder structure, organizing and best practices
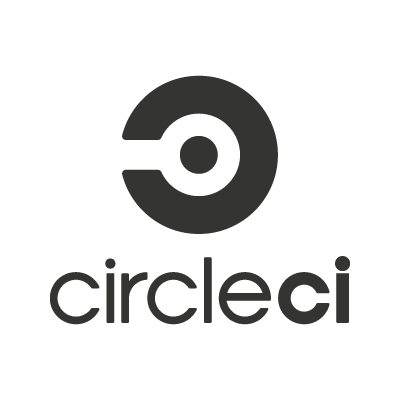
Continuous Integration with Circle CI v2 with Node.js and PHP examples
Continuous integration with Circle CI v2 with examples of code style check in Node.js and PHP.
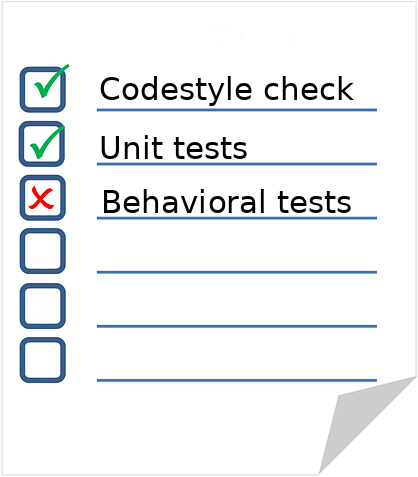
Continuous integration using Travis CI and Behat
Continuous integration using Travis CI and Behat. Building a docker container for running on Travis CI.
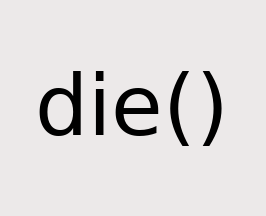
How to find the line on which execution was interrupted in PHP
Example of code to determine the line where the script execution was interrupted
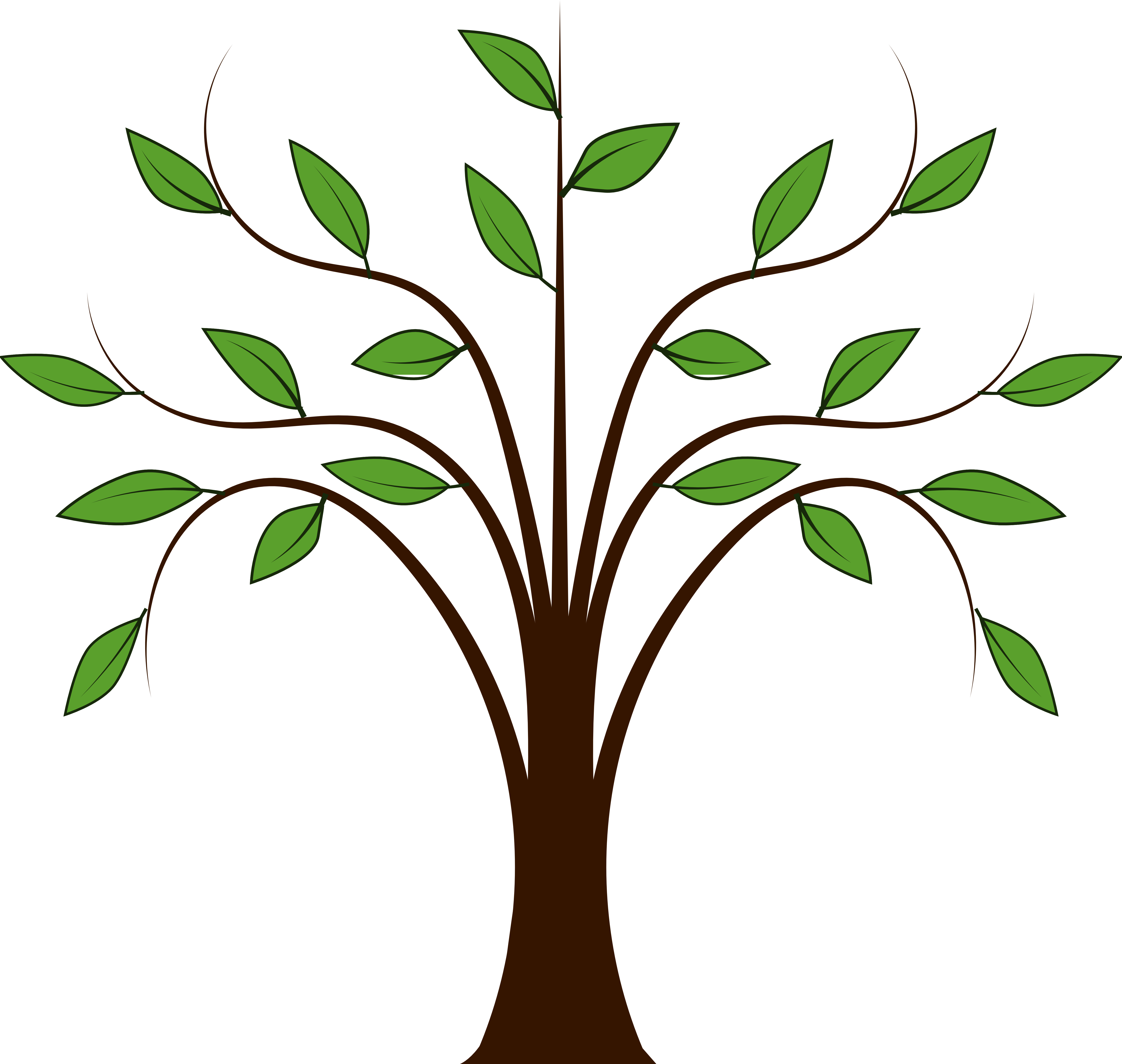
Refreshing Nested Set Tree Indexes in Symfony
Symfony command for recalculating indexes of a Nested Set tree.
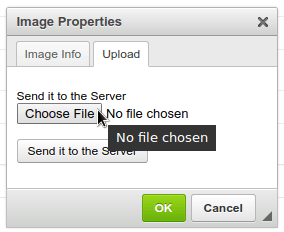
Uploading files in Symfony
Uploading files in Symfony is conceptually no different from other PHP platforms, but still has its own peculiarities due to the presence of additional tools provided by the framework.
Asset versioning using gulp
Asset versioning using gulp with gulp-rev, gulp-rev-replace, and gulp-rename.
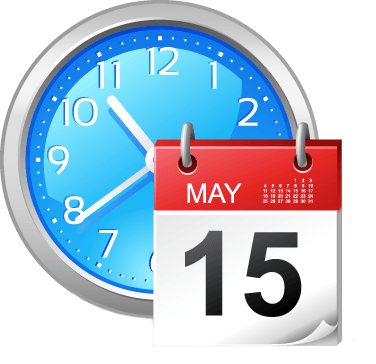
Iteration over a time period in PHP
I have repeatedly had to work in php with a list of dates (by day, for example) if there is a start and end of the period ($dateStart, $dateEnd). For example, in order to synchronize working/weekend days with the backend for the DatePicker from jQueryUI.